ES2023 - New array methods (Part 1)
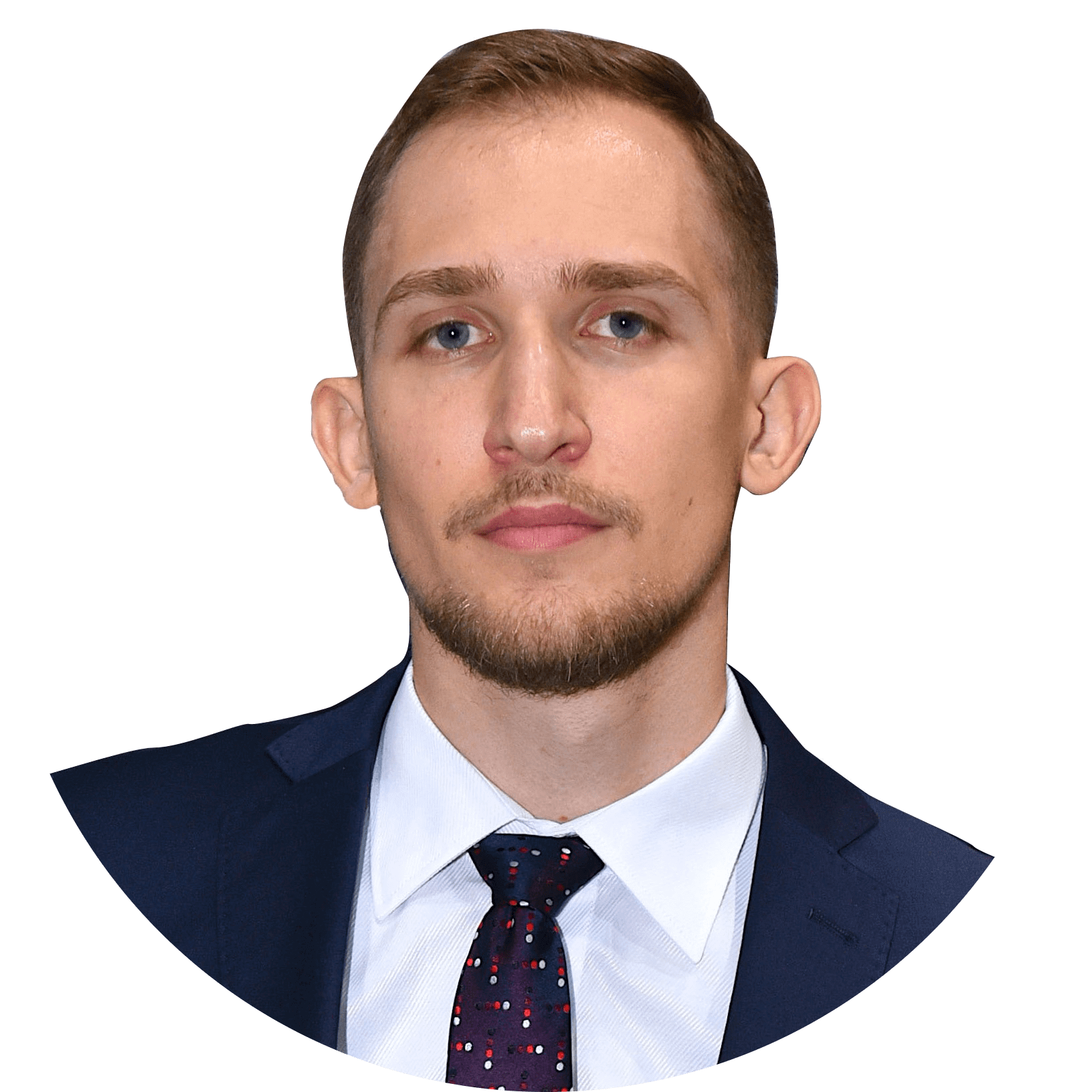
In this mini series of "What's new in ES2023", I'm going to walk you through the finished proposals that are included in the latest draft of the 2023 specification. In the first part, we'll dive into 2 new proposals dealing with arrays, how to use them, in what situations, and also compare them with their predecessors. So lets dive into this :).
Array find from last
No more cloning, reversing and using find anymore. In ECMAScript 2023 there's more cleaner and performant way to find items or indexes occurring at the end of the array. So it essentialy searches from right to left which may comes pretty handy in certain situations.
1. findLast
Before ES2023 you could use .find()
that searches from left to right. So essentialy it loops through the array from start until it finds an item but for larger datasets it would took a while. Next step would be using reverse()
method to change the order of an array and use find again. Since reverse is mutating the underlaying array, a lot of times you would need to create a copy of an array beforehand.
Doing copy, reverse and find seems a lot of work to just find an item. Sure there's a lodash findLast method, but isn't it cool not just to use a native method and don't have to import yet another dependency to your project? :).
Signature of the method Array<T>.prototype.findLast(predicateFn) -> T
.
const array = new Uint8Array([5, 35, 66, 100]);
// Search from left to right
array.find(number => number === 100);
// With reverse:
[...array].reverse().find((number) => number === 100);
// ES2023
array.findLast((number) => number === 100);
Console output:
Checking find(): 5
Checking find(): 35
Checking find(): 66
Checking find(): 100
Number found by find(): 100
Checking findLast(): 100
Number found by findLast(): 100
2. findLastIndex
The same principles apply to .findLastIindex()
. Instead of an item it returns an index.
Signature of the method Array.prototype.findLastIndex(predicateFn) -> number
.
const array = new Uint8Array([5, 35, 66, 100]);
// Before
array.length - 1 - [...array].reverse().findIndex((number) => number === 100);
// ES2023
array.findLastIndex((n) => n === 100)
Change array by copy
Each one of these methods returns a copy of an array instead of mutating it, which can come very handy when dealing with immutable data espeacially immutable state updates in react for example. So no longer manually creating copy before mutating it needed, just use one of these methods :).
3. toReversed
immutable equivalent of reverse()
for reversing the order of an array.
Signature of the method Array.prototype.toReversed() -> Array
.
const array = new Uint8Array([1, 2, 3]);
// Before
[...array].reverse();
// ES2023
array.toReversed();
[3, 2, 1]
4. toSorted
Immutable equivalent of sort()
for sorting and array.
Signature of the method Array.prototype.toSorted(compareFn) -> Array
.
const outOfOrderArr = new Uint8Array([3, 1, 2]);
outOfOrderArr.toSorted()
[1, 2, 3]
5. toSpliced
Immutable equivalent of splice()
for removing n number of items in array determined by start index. In the code example, we removed number 6 from sequence of numbers 1..10, by removing an item at index 5. If we were to remove for example numbers 6 & 7, we would just passed to a second parameter of the method 2
, which referes to number of items to delete.
Signature of the method Array.prototype.toSpliced(start, deleteCount, ...items) -> Array
.
const sequence = [...Array(10).keys()].map((n) => n + 1);
sequence.toSpliced(5, 1);
[1, 2, 3, 4, 5, 7, 8, 9, 10]
6. with
This method makes me super excited, especially because it makes the life more for react developers, who often find themselves in situations where they have to update an item in immutable manner. Essentialy it updates an item in array at certain index and returns a new updated copy of that array.
As you can see from the next example we reduced number of lines from 2 or potentially more to just one liner by using with
method. Isn't it awesome ?
Signature of the method Array.prototype.with(index, value) -> Array
.
const correctionNeeded = [1, 1, 3];
// Before
correctionNeeded.map((num, index) => {
if(index === 1) return 2;
return num;
});
// Or
const temp = [...correctionNeeded];
temp[1] = 2;
// ES2023
correctionNeeded.with(1, 2);
[1, 2, 3]
Compatibility
All of these methods are supported in all modern browsers except firefox, in that case you would need to use polyfill. So if you want to use these methods today, you need to install core-js and import just methods you want to use or use the stable package.
As for typescript users, there are no type definitions for these methods yet, but there is an open PR waiting to be merged. In the meanwhile you have to add those types by yourself.
Summary
In the next part I'll show you how to use these methods in React, because some of them might be pretty helpfull :).